What is a computer system?
A computer system is made up of software, data, hardware, communications and people.
Each computer system can be divided up into a set of sub-systems. Each sub-system can be further divided into sub-systems and so on until each sub-system just performs a single action.
Computer systems can be very large, very small or any size in between.
Examples of computer systems: an app on your smart phone for your alarm clock. The weather forecast that you check on your computer.
The alarm clock is a very small computer system, and the weather forecast is a very large computer system.
Tools and techniques
Top-down design
Top-down design is the breaking down of a computer system into a set of sub-systems, then breaking each sub-system down into a set of smaller sub-systems, until each sub-system just performs a single action.
Structure diagrams
In order to show top-down design in a diagrammatic form, structure diagrams can be used. The structure diagram shows the design of a computer system in a hierarchical way, with each level giving a more detailed breakdown of the system into sub-systems.
Example of an alarm app for a smartphone:
Activity 1
Break down the “check time” sub-system from the smartphone alarm app into further sub-systems.
Activity 2
Draw a structure diagram for cleaning your teeth.
Flowcharts
A flowchart shows diagrammatically the steps required for a task (sub-system) and the order that they are to be performed. These steps together with the order are called an algorithm.
Example of a flowchart for the “checking for the alarm time sub-system”.
Pseudocode
Pseudocode is a simple method of showing an algorithm, using English-like words and mathematical operators that are set out to look like a program.
Pseudocode example for the “checking for the alarm time algorithm”.
REPEAT
Wait 10 seconds
Get Time
UNTIL Time = Alarm Time
Sound Alarm
Library routines
A library routine is a set of programming instructions for a given task that is already available for use. It is already well tested and performs a task that is frequently required. For example, the task “get time” in the “checking for the alarm time” algorithm would probably be readily available as a library routine.
Sub-routines
A sub-routine is a set of programming instructions for a given task that forms a sub-system, not the whole system. Sub-routines written in high-level programming languages are called ‘procedures’ or ‘functions’ depending on how they are used.
Algorithms
An algorithm are all the steps needed to solve a problem. An algorithm is usually represented as a flowchat or pseudocode.
Trace tables
A trace table is used to record the results from each step in an algorithm; it is used to record the value of a variable each time it changes. This manual exercise is called a dry run. A trace table is set up with a column for each variable and a column for any output.
Activity 3
Dry run the following algorithm using a trace table and these test data: 9, 7, 3, 12, 6, 4, 15, 2, 8, 5.
Trace table:
Identifying and correcting errors
Activity 4
Use a trace table and the test data 400, 800, 190, 170, 300, 110, 600, 150, 130, 900 to record another dry run of the pseudocode or flowchart.
If your dry run is correct you should have identified an error. What is the error?
The following is a much better algorithm. Find out why using a trace table and a set test data.
Test data
In order to determine whether a solution is working as it should, it needs to be tested.
A set of test data is all the items of data required to work through a solution.
Validation
Validation is one way of trying to reduce the number of errors in the data being entered into your system.
Validation aims to make sure that data is sensible, reasonable, complete and within acceptable boundaries.
Types of validation
Presence check. A presence check makes sure that a critical field cannot be left blank, it must be filled in. If someone tries to leave the field blank then an error message will appear and you won't be able to progress to another record or save any other data which you have entered.
Range check. Ensures that data is within a defined range. A range check is commonly used when you are working with data which consists of numbers, currency or dates/times.
Testing with normal, extreme and abnormal data. Imagine we are testing a text box to make sure that it will only accept entries of numbers between 1 and 5. The test data would look like this:
Type check. A type check will ensure that the correct type of data is entered into that field. For example, in a clothes shop, dress sizes may range from 8 to 18. A number data type would be a suitable choice for this data. By setting the data type as a number, only numbers could be entered e.g. 10, 12, 14 and you would prevent anyone trying to enter text such as ‘ten’ or ‘ten and a half’.
Length check. Sometimes you may have a set of data which always has the same number of characters. For example, a Spanish mobile number has 9 numbers.
Format check. Some types of data will always consist of the same pattern.
Example 1
Think about a postcode. The majority of postcodes look something like this:
CV36 7TP
WR14 5WB
Replace either of those examples with L for any letter which appears and N for any number that appears and you will end up with:
LLNN NLL
This means that you can set up a format check for something like a postcode field to ensure that a letter isn't entered where a number should be or a number in place of a letter.
Example 2
A National Insurance number must be in the form of XX 99 99 99 X. The first two and the last characters must be letters. The other six characters are numbers. Any format entered differently to this will be rejected.
Check digit. This is used when you want to be sure that a range of numbers has been entered correctly.
Algorithm for calculating the check digit:
1. Add all the digits in even numbered positions together.
2. Multiply the result above by 3.
3. Add all the digits in odd numbered positions together.
4. Add results (2) and (3) together.
5. Calculate the remainder (modulo 10) of result (4).
6. Subtract (5) above from 10
Exercise 1:
Calculate the check digit of the following ISBN (International Standard Book Number):
9 7 8 0 9 5 7 3 4 0 4 1 - 1
where the last one is the check digit.
Different types of check may be used on the same piece of data; for example, an examination mark could be checked for reasonableness by using a range check, a type check and a presence check. When data is validated by a computer system, if the data is rejected a message should be output explaining why the data was rejected and another opportunity given to enter the data.
Verification
Verification is checking that data has been accurately copied onto the computer or transferred from one part of a computer system to another.
Verification methods include:
Double entry.
Screen/visual check.
Parity check.
Checksum.
For double entry the data is entered twice, sometimes by different operators; the computer system compares both entries and outputs an error message requesting that the data is entered again if they are different.
A screen/visual check is a manual check completed by the user who is entering the data. When the data entry is complete the data is displayed on the screen and the user is asked to confirm that it is correct before continuing. The user checks the data on the screen against a paper document that is being used as an input form or confirms from their own knowledge if the data is about them.
Creating algorithms
Exercises
Create a flowchart that allows you to enter all your subject scores and outputs your average.
Modify the above flowchart so it validates the scores entered. For example, if the user enters a number smaller than 0 or greater than 100 the program should reject the entry.
Daniel lives in Italy and travels to Mexico, India and New Zealand. The time differences are:
Thus, if it is 10:15 in Italy it will be 14:45 in India.
a) Write an algorithm, using pseudocode or otherwise, which:
inputs the name of the country.
inputs the time in Italy in hours (H) and minutes (M).
calculates the time in the country input using the data from the table.
outputs the country and the time in hours and minutes.
Pseudocode
Assignment
Values are assigned to a variable using the arrow operator: ←
Examples:
Cost ← 10
Price ← Cost * 2
Tax ← Price * 0.12
SellingPrice ← Price + Tax
Gender ← “M”
Chosen ← False
What are the values of Price and SellingPrice?
What are the data types of Cost, Price, Tax, SellingPrice, Gender, Chosen?
Conditional statements
They are used to decide between different actions to be taken by an algorithm according to the values of the variables.
IF. It is a condition that can be true or false. The THEN path is followed if the condition is true and the ELSE path is followed if the condition is false. There may or may not be and ELSE path. The end of the statement is shown by ENDIF.
A condition can be set up in different ways:
Using a Boolean variable that can have the value TRUE or FALSE. Example:
IF Found
THEN PRINT “Your search was successful.”
ELSE PRINT “Your search was unsuccessful.”
ENDIF
Using comparison operators.
IF Age < 18
THEN PRINT “Child”
ELSE PRINT “Adult”
ENDIF
Comparison operators:
> greater than
< less than
= equal
>= greater than or equal
<= less than or equal
<> not equal
AND both
OR either
NOT not
Exercise:
Create an algorithm in pseudocode that checks if a percentage mark input by the user is valid. And, if it is valid outputs if it is a Pass or a Fail.
CASE
For a CASE condition the value of the variable decides the path to be taken.
CASE Grade OF
“a” : PRINT “Excellent”
“b” : PRINT “Good”
“c” : PRINT “Acceptable”
“d” : PRINT “Poor”
OTHERWISE PRINT “Improvement is needed”
ENDCASE
Exercise: Use a CASE statement to display the day of the week if the variable DAY has the value 1 to 7 and an error otherwise.
INPUT and OUTPUT
INPUT and OUTPUT are used for the entry of data and display of information. Sometimes READ can be used instead of INPUT. You might also find, PRINT is used instead of OUTPUT.
Loop structures. When some actions performed as part of an algorithm need repeating, this is called “iteration”. Loop structures are used to perform the iteration.
Types of loop structures:
FOR … TO … NEXT
A variable is set up with a start value and an end value and then incremented in steps of one until the end value is reached and the iteration finishes.
Example: print an asterisk (*) ten times.
FOR i ← 1 TO 10
PRINT “*”
NEXT
REPEAT … UNTIL
This loop structure is used when the number of iterations is not known and the actions are repeated UNTIL a given condition becomes true.
Example: ask the user to enter a number between 1 and 10. If the user enters a number outside this range ask him/her to enter a number again until the number is inside this range.
WHILE … DO … ENDWHILE
This loop structure is used when the number of repetitions is not known and the actions are only repeated WHILE a given condition is true.
Example: ask the user to enter a number between 1 and 10. If the user enters a number outside this range ask him/her to enter a number again until the number is inside this range.
Exercises
Write a program that prints the numbers from 10 to 20.
Print all even numbers smaller than 100.
Write a program to add numbers until user enters zero.
Ask the user for 2 numbers and then ask if he/she wants to add, multiply, subtract or divide these 2 numbers.
Review of flowcharts and trace tables
The flowchart below inputs the weight of a number of parcels in kilograms. Parcels weighing more than 25 kilograms are rejected. A value of –1 stops the input. The following information is output: the total weight of the parcels accepted and number of parcels rejected. (0478/21/M/J/15 pg 8 and 9)
(0478/22/M/J/15 pg 6, 7, 8 and 9)
Databases
A database is a structured collection of data that allows people to extract information in a way that meets their needs. The data can include text, numbers, pictures, anything that can be stored in a computer.
Databases are very useful in preventing data problems ocurring because:
Data is only stored once - no data duplication.
If any changes or additions are made, it only has to be done once - the data is consistent.
The same data is used by everyone.
What are databases used for?
To store information about people, things, for example:
Patients in a hospital.
Students in a school.
Cars to be sold.
Books in a library.
Exercise: find more uses for databases, and for each one decide what sort of information is being stored.
The structure of a database
Inside a database, data is stored in tables, which consists of many records and each record consists of several fields.
In order to be sure that each record can be found easily and to prevent duplication of data (the same record being inputted more than once), each record includes a PRIMARY KEY field. Each primary key field in the table is unique.
The primary key can be a field that is already used, provided it is unique, for example the ISBN in a book table, or a new field could be added to each record, for example, an idMovie field was added to the Movies table below.
Sometimes, a primary key can be formed by using two or more existing fields, for example, the doctor’s appointment could have a primary key made from the date and the time of each appointment.
Do you remember the Movies database? The following is the table Movies.
Query-by-example
Example:
In the Movies database the president of the directors wants to be reminded before the first meeting in the month of any director who will have a birthday that month.
The president wants to see the name of any director with a date birth this month.
Exercise:
1 A motor car manufacturer offers various combinations of:
seat colours
seat materials
car paint colours
A database was set up to help customers choose which seat and paint combinations were possible.
a) How many records are shown in the database?
Answer: 10
b) The following search condition was entered using a query-by-example grid:
What will be displayed?
c) A customer wants to know the possible combinations for a car with leather seats and either silver or grey paint.
What search condition needs to be input? Copy and complete the table.
2 A database was set up to compare oil companies. A section of the database is shown below:
a) How many fields are there in each record?
Answer: 7
b) The following search condition was entered:
(No of countries < 30) AND (Head office = “Americas”)
Using Code only, which records would be output?
c) What search condition is needed to find out which oil companies have a share price less than $50 or whose profits were greater than 8 billion dollars?
Arrays
An array is a collection of data that holds a fixed number of values of the same type.
In order to use a one dimensional array in a computer program, you need to consider:
what the array is going to be used for, so it can be given a meaningful name.
how many items are going to be stored, so the size of the array can be determined.
what sort of data is to be stored, so that the array can be the appropriate data type.
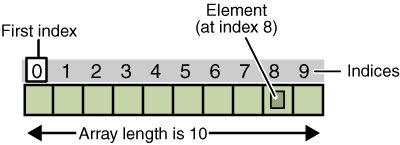
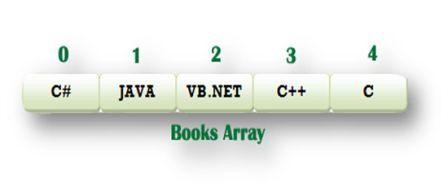
Pseudocode to fill in an empty array:
DECLARE A[1:10] OF STRING
FOR i ← 0 TO 9
INPUT fruit
A[i] ← fruit
NEXT
Pseudocode to print the contents of an array:
FOR i ← 0 TO 9
PRINT A[i]
NEXT
Exercises
Declare 3 arrays to store:
The names of 5 students.
The gender of each student as M or F.
The Computer Science marks of these five students.
Write a program that will allow you to enter all the data mentioned in question 1.
Add validation checks. For example, when inputting the gender only M or F should be allowed. When entering the marks only numbers from 0 to 100 should be allowed.
Find the average mark of the 5 students.
Find the student with the highest mark. Output the student’s name and mark.
Find the student with the lowest mark. Output the student’s name and mark.
Find the number of male and female students. Output these numbers with an appropriate message.
Python Programming - Arrays
For Monday, 17/02/2020:
Write a program in pseudocode and Python that finds the largest number in an array. For example:
If you have the array:
MyArray = [89, 14, 90, 5, 2, 66]
Your program should output:
The largest number in the array is: 90
Your program should work for any array.
Note: when I test your program I will NOT use the array in this example. I will set a different array.